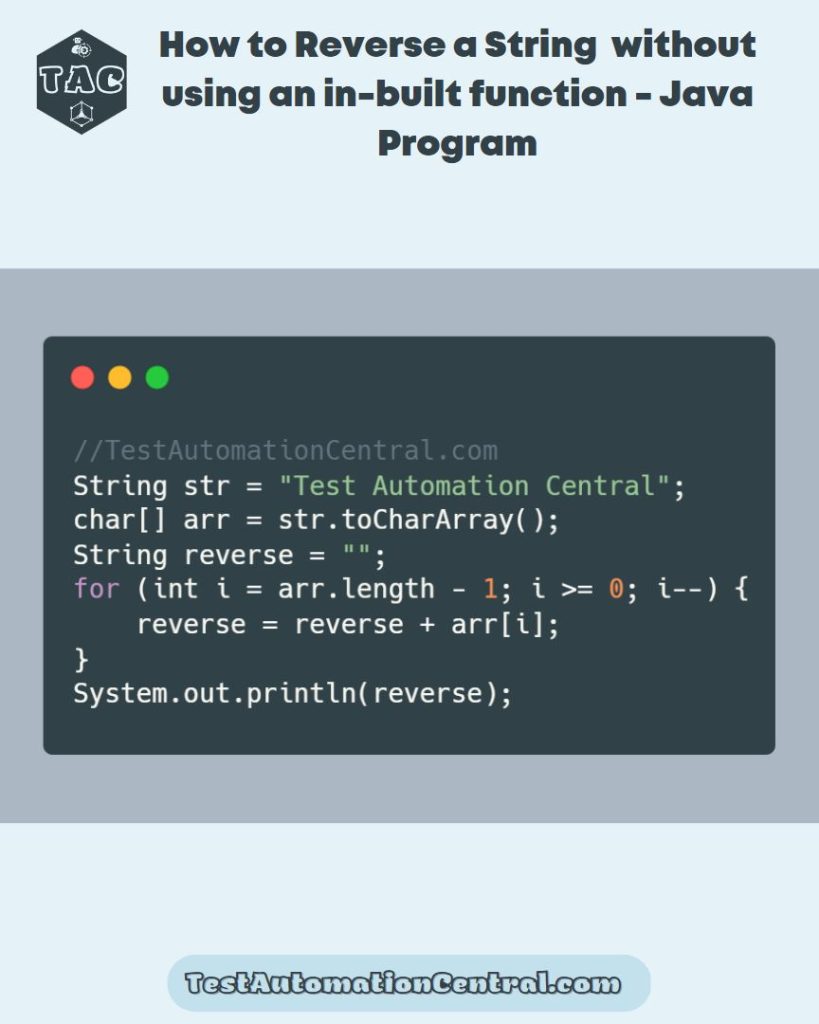
Java Programs on String are commonly asked interview questions. In this article, we will discuss – how to reverse a String in Java without using an in-built function.
Step 1: First, we will convert the String to a char[] array using String function – toCharArray() and store it in variable arr
Step 2: Initialized an empty string i.e. null
Step 3: Write a for loop which adds each character in reverse order – reverse = reverse + arr[i];
Example – Code Snippet:
package TestAutomationCentral; public class ReverseString { public static void main(String[] args) { //TestAutomationCentral.com String str = "Test Automation Central"; char[] arr = str.toCharArray(); String reverse = ""; for (int i = arr.length - 1; i >= 0; i--) { reverse = reverse + arr[i]; } System.out.println(reverse); } }