In this tutorial, we will learn how to find the index of a specific element in an array using Java. These questions will be asked in the Java Interview and here we have provided detailed tutorials on how to find the Array index of a specific element.
Steps:
- To find the index of element 2, we will write a method findIndex(int[] arr, int value)
- We can use a loop to iterate over the array and compare each element with the target element to find the index of a specific element in an array.
- If we find a match, we can return the index of that element. If we do not find a match, we can return -1 to indicate that the element is not present in the array.
- Call findIndex() method in the main by passing the required parameters
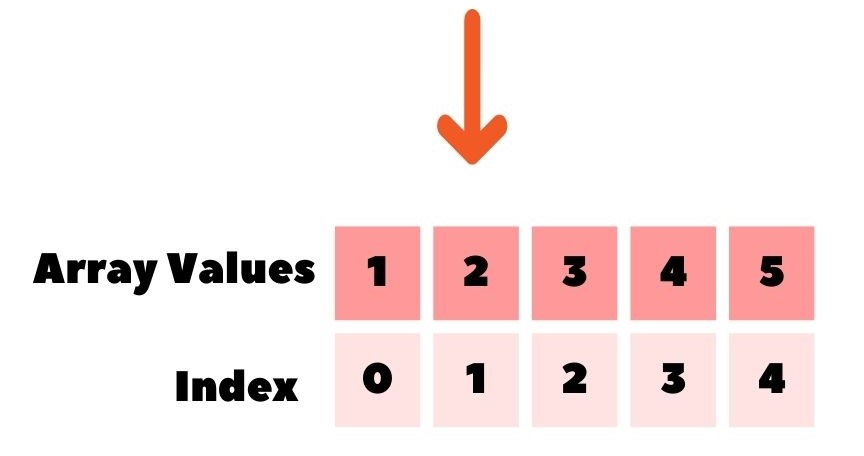
Java Program to Find the Index of a Specific Element in an Array
package TestAutomationCentral;
public class FindIndexOfArray {
public static void main(String[] args) {
//TestAutomationCentral.com
int[] arr = {1, 2, 3, 4, 5};
System.out.println(findIndex(arr, 2));
}
public static int findIndex(int[] arr, int value) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == value) {
return i;
}
}
return -1;
}
}
Output:
1